[SPRING_입문]/개발일지
[2주차] 개발일지 (2-5) 생성일자, 수정일자
Code_Otaku
2022. 6. 16. 16:38
1. 상속 (Inheritance)
뜬금없이 왠 상속개념이냐고?
단순히 JPA 뿐만 아니라 상속은 JAVA에서 코드의 재사용율을 높이기 위한 가장 핵심적인 기능이다.
거두절미하고 코드 먼저 살펴보자.
class Person {
private String name;
private String getName() {
return this.name;
}
}
class Tutor extends Person {
private String address;
// Person 클래스를 상속했기 때문에,
// name 멤버변수와 getName() 메소드를 가지고 있습니다.
}
Tutor 클래스는 Person 클래스를 상속한다.
즉, Person 클래스는 Tutor 클래스의 부모가 되는 셈이다.
자식 클래스가 되는 Tutor 클래스는 부모 클래스의 메서드를 마음껏 가져다가 쓸 수 있다.
단, 반대의 경우는 성립되지 않는다.
그리고 부모 클래스는 수많은 자식 클래스들을 거느리게 되는데
자바에서는 형제 클래스라는 개념이 없다.
이 점도 유의하고 넘어가자.
2. 생성일자 / 수정일자
데이터베이스의 핵심적인 기능은 뭐니뭐니해도 날짜 전달일 것이다.
JPA에는 SQL문을 직접 수정하지 않고도 날짜를 생성해주는 기능이 있다.
다음 코드를 참조할 것!
package com.sparta.week02.domain;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedDate;
import org.springframework.data.jpa.domain.support.AuditingEntityListener;
import javax.persistence.EntityListeners;
import javax.persistence.MappedSuperclass;
import java.time.LocalDateTime;
@MappedSuperclass // 상속했을 때, 컬럼으로 인식하게 합니다.
@EntityListeners(AuditingEntityListener.class) // 생성/수정 시간을 자동으로 반영하도록 설정
public abstract class Timestamped {
@CreatedDate // 생성일자임을 나타냅니다.
private LocalDateTime createdAt;
@LastModifiedDate // 마지막 수정일자임을 나타냅니다.
private LocalDateTime modifiedAt;
}
package com.sparta.week02.domain;
import lombok.NoArgsConstructor;
import javax.persistence.*;
@NoArgsConstructor // 기본생성자를 대신 생성해줍니다.
@Entity // 테이블임을 나타냅니다.
public class Course extends Timestamped{
// Course 클래스는 Timestamped 클래스를 상속해서 쓴다.
// Timestamped 클래스는 추상 클래스
@Id // ID 값, Primary Key로 사용하겠다는 뜻입니다.
@GeneratedValue(strategy = GenerationType.AUTO) // 자동 증가 명령입니다.
private Long id;
@Column(nullable = false) // 컬럼 값이고 반드시 값이 존재해야 함을 나타냅니다.
private String title;
@Column(nullable = false)
private String tutor;
public String getTitle() {
return this.title;
}
public String getTutor() {
return this.tutor;
}
public Course(String title, String tutor) {
this.title = title;
this.tutor = tutor;
}
}
코드 주석과 함께 상속 관계를 잘 살펴보아야 할 것이다.
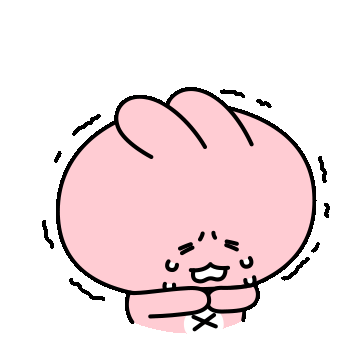